Overview
Enabling Statamic's Git integration is like having Spock in your enterprise, listening for content changes with those large handsome ears. You won't find anyone more committed. 🖖
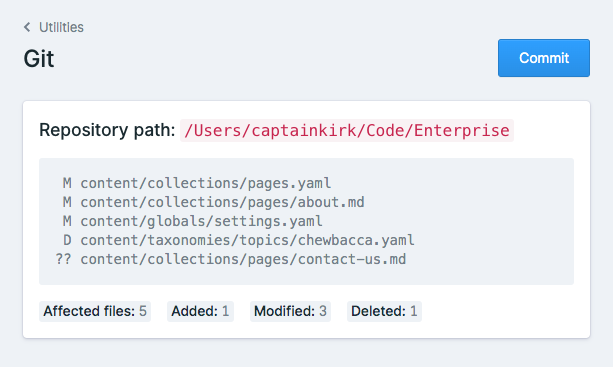
Enabling
To enable in a specific environment, add the following to your .env
file:
STATAMIC_GIT_ENABLED=true
By default, content will be committed automatically as it's changed, but you can customize your git workflow using the provided configuration options.
Configuration
Git workflow can be configured in your config/statamic/git.php
file, or per environment in your .env
file.
Git User
By default, Statamic will attempt to use the authenticated user's name and email when committing changes. If you prefer to always use hardcoded git user info, you can disable this by setting use_authenticated
to false
in your configuration:
'use_authenticated' => true, 'user' => [ 'name' => env('STATAMIC_GIT_USER_NAME', 'Spock'),],
Note: Depending on how you configure your commit workflow, an authenticated user may not always be available. In these cases, Statamic will fall back to the above configured user.
Tracked Paths
You are free to define the tracked paths to be considered when staging and committing changes. Default stache and file locations are already set up for you, but feel free to modify these paths in your configuration to suit your storage config.
'paths' => [ base_path('content'), base_path('users'), resource_path('blueprints'), resource_path('fieldsets'), resource_path('forms'), resource_path('users'), storage_path('forms'), public_path('assets'),],
You may also reference absolute paths to external repositories! If Statamic detects an external repository path, changes will be staged and committed relative to your external repository.
Committing Changes
By default, Statamic listens to various Saved
and Deleted
data events to determine when your content is changed, and will automatically commit your changes. If you prefer users to manually trigger commits using the Git utility interface, you may set this to false
in your configuration:
'automatic' => env('STATAMIC_GIT_AUTOMATIC', false),
Or in a specific environment's .env
file:
STATAMIC_GIT_AUTOMATIC=false
From the command line
Manually trigger commits via the command line with the following command:
php please git:commit
Pushing Changes
Statamic can also git push
your changes after committing. Enable this behavior in your configuration:
'push' => env('STATAMIC_GIT_PUSH', true)
Or in a specific environment's .env
file:
STATAMIC_GIT_PUSH=true
Remote setup
When pushing, Statamic assumes you have a Git remote with an upstream branch set, and are authenticated to push to your remote via SSH.
If you use Laravel Forge or Ploi to deploy your site, a git remote and upstream branch will automatically be configured for you.
If a remote and upstream is not already configured for your deployment, you may need to set this up manually on your server. For example, the following commands could be run from your deployment folder to add an origin
remote and track the master
branch:
git initgit fetchgit branch --track master origin/mastergit reset HEAD
Queueing Commits
When automatic committing is enabled, commits are automatically pushed onto a queue for processing. By default, your Statamic app is configured to use the sync
queue driver, which will run the job immediately after your content is saved during the web request.
QUEUE_CONNECTION=sync
Queueing for performance
If you are experiencing slow-down when saving or deleting content in the control panel, we recommended configuring another queue driver so that commits can be run by a background process, which will help keep the experience fast for your users.
A popular choice is to use a Redis store and queue driver, along with Laravel Horizon for managing your Redis queues.
Note: When commits are run by a queue's background process, there will be no authenticated user. In this case, Statamic will use the hardcoded git user in your configuration.
Delaying Commits
When queueing commits, you can also configure a dispatch delay for your commits:
'dispatch_delay' => env('STATAMIC_GIT_DISPATCH_DELAY', 10),
Or in a specific environment's .env
file:
STATAMIC_GIT_DISPATCH_DELAY=10
In this example, we queue a delayed commit to run 10 minutes after a user makes a content change. If at that time the repository status is clean, the commit will be cancelled. Please note that the default sync
queue driver does not support this. Use another queue driver like redis
instead.
Since all tracked paths are committed at once, this can allow for more consolidated commits when you have multiple users making simultaneous content changes to your repository.
Note: When commits are run by a queue's background process, there will be no authenticated user. In this case, Statamic will use the hardcoded git user in your configuration.
Scheduling Commits
You can also schedule commits to run via cron job at regular intervals within your app/Console/Kernel.php
file:
protected function schedule(Schedule $schedule){ $schedule ->command('statamic:git:commit') ->everyTenMinutes();}
In this example, we schedule a commit to run 10 minutes after a user makes a content change. If at that time the repository status is clean, the commit will be cancelled.
Note: If you have never used Laravel's scheduler, be sure to also configure a cron job on your server to run all scheduled jobs. This only needs to be done once per server.
* * * * * cd /path-to-your-project && php artisan schedule:run >> /dev/null 2>&1
Scheduling for performance
Scheduling commits can be a great alternative to queueing, for when you don't have a proper queue setup. If you choose to schedule commits, just be sure to disable automatic commit functionality as well.
Since all tracked paths are committed at once, this can allow for more consolidated commits when you have multiple users making simultaneous content changes to your repository.
Note: When commits are scheduled to run via cron, there will be no authenticated user. In this case, Statamic will use the hardcoded git user in your configuration.
Customizing Commits
To customize the commit messages themselves, modify the commands
array in the configuration file.
For example, you can append [BOT]
to the commit message so that you can selectively disable automatic site deployments when a commit is automatically pushed back to your repository:
'commands' => [ 'git add {{ paths }}', 'git -c "user.name={{ name }}" -c "user.email={{ email }}" commit -m "{{ message }} [BOT]"',],
In your deploy scripts on Forge, Ploi, or Cleavr you could then add the following:
Forge
if [[ $FORGE_DEPLOY_MESSAGE =~ "[BOT]" ]]; then echo "AUTO-COMMITTED ON PRODUCTION. NOTHING TO DEPLOY." exit 0fi
Ploi
if [[ {COMMIT_MESSAGE} =~ "[BOT]" ]]; then echo "AUTO-COMMITTED ON PRODUCTION. NOTHING TO DEPLOY." exit 0fi
Cleavr
if [[ "{{ commitMessage }}" =~ "[BOT]" ]]; then echo 'AUTO-COMMITTED ON PRODUCTION. NOTHING TO DEPLOY.' exit 1fi
Ignoring Events
When automatically committing, Statamic will listen on all Saved
and Deleted
events, as well as any events registered by installed addons. To ignore specific events, add them to the ignored_events
array in the configuration file.
For example, if you're storing users in a database, you may wish to ignore user-based events:
'ignored_events' => [ \Statamic\Events\Data\UserSaved::class, \Statamic\Events\Data\UserDeleted::class,],
When ignoring events, you may also wish to remove any related tracked paths from your configuration.
Addon Events
When building an addon that provides its own content saved events, you should register those events with our git listener in your addon service provider:
\Statamic\Facades\Git::listen(PunSaved::class);
This will allow your end users to track addon-related content in their tracked paths, if they decide to opt-in for automatic commit and push when saving.
Providing a default commit message
You can also provide a default commit message by implementing the ProvidesCommitMessage
interface with a commitMessage()
method definition:
<?php namespace Punner\Events; use Statamic\Contracts\Git\ProvidesCommitMessage;use Statamic\Events\Event; class PunSaved extends Event implements ProvidesCommitMessage{ public $item; public function __construct($item) { $this->item = $item; } public function commitMessage() { return __('Pun saved'); }}