Taxonomy Term Repository
To work with the Repository Term queries, use the following Facade:
use Statamic\Facades\Term;
Methods
Methods | Description |
---|---|
all() |
Get all Terms |
find($id) |
Get Term by id |
findByUri($uri) |
Get Term by uri |
findOrFail($id) |
Get Term by id . Throws a TermNotFoundException when the term cannot be found. |
query() |
Query Builder |
make() |
Makes a new Term instance |
Querying
Examples
Get a single term by its id
When getting a single term by its ID, the value of the $id
parameter should be taxonomy_handle::term_id
.
Term::query()->where('id', 'tags::123')->first(); // Or with the shorthand methodTerm::find('tags::123');
When a term can't be found, the Term::find()
method will return null
. If you'd prefer an exception be thrown, you may use the findOrFail
method:
Term::findOrFail('tags::123');
Get all tags
Term::query() ->where('taxonomy', 'tags') ->get();
Get all tags in a collection
Term::query() ->where('collection', 'blog') ->where('taxonomy', 'tags') ->get();
Get all tags in multiple collections
Term::query() ->where('taxonomy', 'tags') ->whereIn('collection', ['blog', 'news']) ->get();
Only include tags attached to entries
Term::query() ->where('taxonomy', 'tags') ->where('entries_count', '>=', 1); ->get();
Creating
Start by making an instance of a term with the make
method.
You need at least a slug and the taxonomy.
$term = Term::make()->taxonomy('tags')->slug('my-term');
Data for a term is stored on a per site basis, even if you only are using a single site.
The method expects a site handle and an array of key-value pairs.
// Example for a single site$term->dataForLocale('default', [ 'mandalorian_code' => 'This Is The Value', //...]);
When using multi-site, you can pass different data to each site.
$term->dataForLocale('default', $data);$term->dataForLocale('fr', $frenchData);
You may call additional methods on the term to customize it further.
$term->blueprint('tag');
Finally, save it. It'll return a boolean for whether it succeeded.
$term->save(); // true or false
Docs Feedback
Submit improvements, related content, or suggestions through Github.
Betterify this page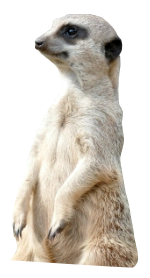