User Repository
To work with the User Repository, use the following Facade:
use Statamic\Facades\User;
Methods
Methods | Description |
---|---|
all() |
Get all Users |
current() |
Get current User |
find($id) |
Get User by id |
findByEmail($email) |
Get User by email |
findByOAuthID($provider, $id) |
Get User by an ID from an OAuth provider |
findOrFail($id) |
Get User by id . Throws a UserNotFoundException when the user cannot be found. |
query() |
Query Builder |
make() |
Makes a new User instance |
Querying
Get a user by ID
User::query() ->where('id', 'abc123') ->first(); // Or with the shorthand methodUser::find('abc123');
When a user can't be found, the User::find()
method will return null
. If you'd prefer an exception be thrown, you may use the findOrFail
method:
User::findOrFail('abc123');
Get a user by email
User::query() ->first(); // Or with the shorthand method
Get a user by OAuth ID
User::findByOAuthId('github', '123');
Get all super users
User::query()->where('super', true)->get();
Get the currently logged in user
User::current();
Creating
Start by making an instance of a user with the make
method.
You need at least an email before you can save a user.
You may call additional methods on the user to customize it further.
$user ->password('plaintext') // it will be hashed for you ->data(['foo' => 'bar']) // an array of data (front-matter) ->preferences($prefs) // array of preferences ->roles($roles) // array of roles ->groups($groups); // array of groups
Finally, save it.
$user->save();
Roles & Groups
In the example above, it demonstrates passing roles & groups as arrays to the ->roles()
and ->groups()
methods.
However, Statamic also provides a few convenience methods for assigning/removing/checking individual roles & groups:
$user->roles(); // Returns a collection of the user's roles$user->roles(['role_1', 'role_2']); // Sets the user's roles (overrides any existing roles)$user->assignRole('role_1'); // Assigns a role to the user$user->removeRole('role_1'); // Removes a role from the user$user->hasRole('role_2'); // Checks if the user has the provided role. $user->groups(); // Returns a collection of the user's groups$user->groups(['group_1', 'group_2']); // Sets the user's groups (overrides any existing groups)$user->addToGroup('group_1'); // Adds the user to a group$user->removeFromGroup('group_1'); // Removes the user from a group$user->isInGroup('group_2'); // Checks if the user is part of a group
Docs Feedback
Submit improvements, related content, or suggestions through Github.
Betterify this page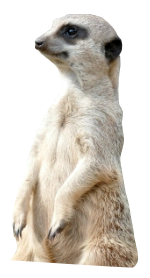