Collection Repository
To work with the with Collection
Repository, use the following Facade:
use Statamic\Facades\Collection;
Methods
Methods | Description |
---|---|
all() |
Get all Collections |
find($id) |
Get Collection by id |
findByHandle($handle) |
Get Collection by handle |
findByMount($mount) |
Get Collection by mounted entry id |
findOrFail($id) |
Get Collection by id . Throws a CollectionNotFoundException when the collection cannot be found. |
handleExists($handle) |
Check to see if Collection exists |
handles() |
Get all Collection handles |
queryEntries() |
Query Builder for Entries |
whereStructured() |
Get all Structured Collections |
make() |
Makes a new Collection instance |
The id
is the same as handle
while using the default Stache driver.
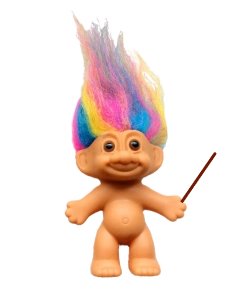
Querying
While the Collection
Repository does not have a Query Builder, you can still query for Entries inside Collections with the queryEntries
method. This approach can be useful for retrieving Entries with an existing Collection object.
$blog = Collection::find('blog'); $blog->queryEntries()->get();
When a collection can't be found, the Collection::find()
method will return null
. If you'd prefer an exception be thrown, you may use the findOrFail
method:
Collection::findOrFail('blog');
Creating
Start by making an instance of a collection with the make
method. You can pass the handle into it.
$collection = Collection::make('assets');
You may call additional methods on the collection to customize it further.
$collection ->title('Blog') ->routes('/blog/{slug}') // a string, or array of strings per site ->mount('blog-page') // id of an entry ->dated(true) ->ampable(false) ->sites(['one', 'two']) // array of handles ->template('template') ->layout('layout') ->cascade($data) // an array ->searchIndex('blog') // index name ->revisionsEnabled(false) ->defaultPublishState('published') // or 'draft' ->structureContents($structure) // an array ->sortField('field') // the field to sort by default ->sortDirection('asc') // or 'desc' ->taxonomies(['tags']) // array of handles ->propagate(true); // whether newly created entries propagate to other sites
Finally, save it.
$collection->save();
Docs Feedback
Submit improvements, related content, or suggestions through Github.
Betterify this page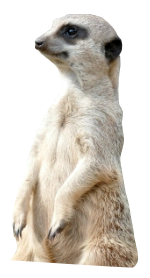